Precision-crafted, React Dropzone component is a standout for drag-and-drop uploads. Lightweight and feature-rich, it's designed for both out-of-the-box elegance and personal customization.
Customizable React Dropzone Component
Unleash the power of a sleek React Dropzone: feather-light, fully style-adaptable with built-in image viewing. Experience effortless file validation and a developer-friendly API for unmatched flexibility.
Quick Start
npm install @zbarakzai/dropzone
Neubulazone
Effortlessly integrate and test our Dropzone component, ensuring smooth file uploads in any React application.
import { DropZone } from "nebulazone";
function DropZoneExample() {
const [files, setFiles] = useState<File[]>([]);
const onDrop = useCallback(
(
files: File[],
acceptedFiles: File[],
rejectedFiles: File[],
errors: ValidationError[],
) => {
setFiles(acceptedFiles);
},
[],
);
return (
<DropZone
accept="image/*"
className="relative border-dashed rounded-md"
onDrop={onDrop}
/>
);
}
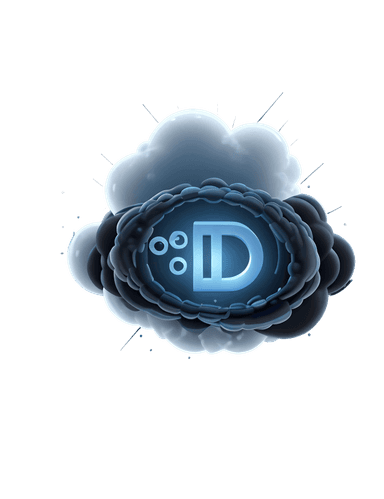
First-class developer experience
Experience a top-tier React dropzone component: fully customizable, with built-in error handling and validation for file size and type.
Props
- Name
accept? string
- Type
- Description
Allowed file types image/* | video/* | text/* | application/zip etc.
- Name
className? string
- Type
- Description
The classess you can add.
- Name
allowMultiple? boolean
- Type
- Description
Can handle multiple file uploads in one go.
- Name
maxFileSize? `${number}${ByteUnits}`
- Type
- Description
The maximum allowed file size 4MB | 2KB ect.
- Name
minFileSize? `${number}${ByteUnits}`
- Type
- Description
The minimum allowed file size 4MB | 2KB ect.
- Name
maxTotalFileSize? `${number}${ByteUnits}`
- Type
- Description
The maximum allowed total file size 1GB etc.
- Name
disabled? boolean;
- Type
- Description
Enables the disabled state.
- Name
children? React.ReactNode;
- Type
- Description
The elements that will be rendered as children inside the dropzone.
- Name
onClick?(event: React.MouseEvent<HTMLElement>, inputRef: React.RefObject<HTMLInputElement>) void
- Type
- Description
Callback invoked on click action.
- Name
onDropAccepted? (acceptedFiles: File[]) => void
- Type
- Description
Callback function that activates when the drop operation includes at least one accepted file.
- Name
onDropRejected? (rejectedFiles: File[]) => void
- Type
- Description
Callback function triggered when the drop operation includes at least one file that was rejected.
- Name
onDragOver? () => void
- Type
- Description
Callback triggered during file drag over the designated area.
- Name
onDragEnter? () => void
- Type
- Description
Callback triggered upon the entry of one or more files into the drag area.
- Name
onDragLeave? () => void
- Type
- Description
Callback triggered when one or more files exit the drag area.
- Name
onDrop? (files: File[], acceptedFiles: File[], rejectedFiles: File[], errors: ValidationError[],) => void
- Type
- Description
Callback triggered when files are droped.
- Name
setError? React.Dispatch<React.SetStateAction<boolean>>
- Type
- Description
Set error true when the internal hasError changes value.
- Name
setFileEnterZone? React.Dispatch<React.SetStateAction<boolean>>
- Type
- Description
Callback to update the state indicating whether a file has entered the drop zone area.
<DropZone
accept='image/*'
className='flex'
allowMultiple
maxFileSize='2MB'
minFileSize='300KB'
maxTotalFileSize='1GB'
disabled={files.length > 10}
onClick={() => {}}
onDropAccepted={(acceptedFiles: File[]) => {}}
onDropRejected={(rejectedFiles: File[]) => {}}
onDrop={(allFiles: File[], accepted: File[], rejected: File[], error: ValidationError[]) => {}}
onDragEnter={() => {}}
onDragLeave={() => {}}
onDragOver={() => {}}
setError={(err: boolean) => {setError(err)}}
setFileEnterZone={(enter: boolean) => {setEnter(enter)}}
/>
Feature Roadmap
PreviewHub
Effortlessly preview various image formats and play dropped videos in our enhanced Dropzone component
CropCraft
Refine visuals effortlessly; CropCraft offers precise image cropping and resizing tailored for perfection.
EditEase
Refine and redefine with EditEase. Achieve impeccable image edits, rotations, and mirror flips.
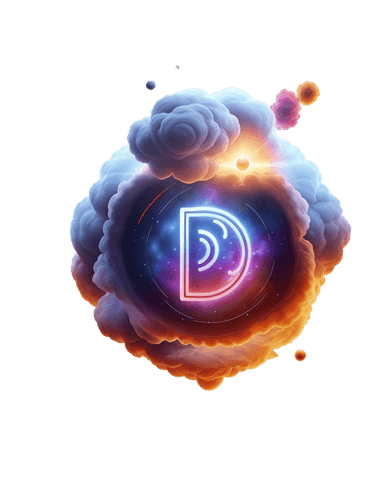
NeubulaZone React Dropzone is my embodiment of a lightweight, user-friendly component, enriched with extensive documentation and examples. Proudly open-sourced on GitHub for community contributions.